Power Automate is a powerful tool for automating workflows, and its ability to handle string manipulation is a critical feature for data transformation, dynamic content generation, and process optimization. In this blog, we’ll explore advanced string manipulation techniques, including splitting strings, conditional string processing, and nested expressions, to handle complex use cases with ease.
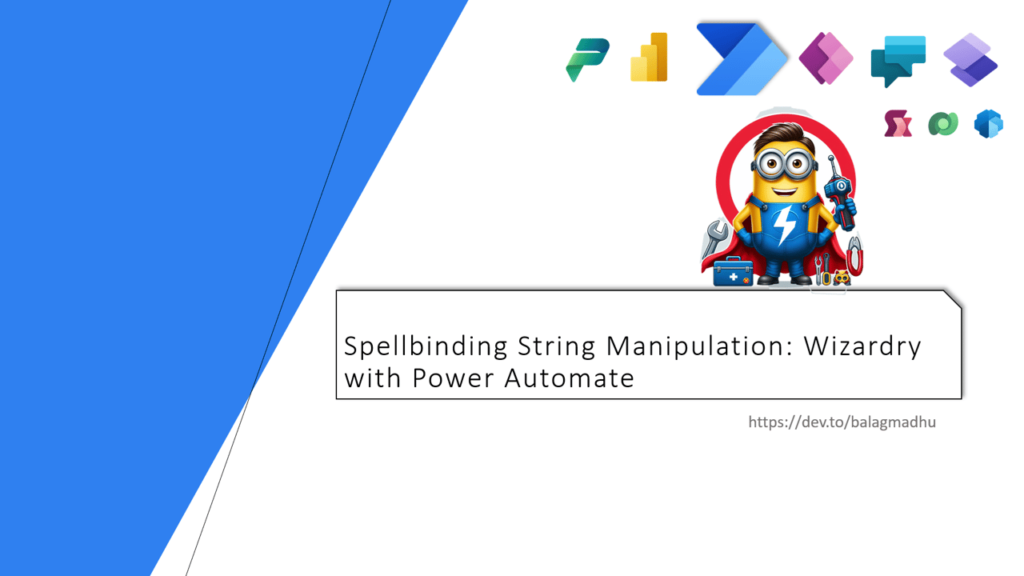
1. Splitting Strings: Breaking Down Complex Data
When dealing with structured data, such as CSVs or strings with delimiters, the split()
function is indispensable. It allows you to divide a string into an array of substrings based on a specified delimiter.
Example: Extracting Data from a CSV Field
Imagine you have a string "John, Doe, john.doe@example.com"
and you want to extract the email address.
Steps:
- Use the
split()
function:powerautomateCopy codesplit('John, Doe, john.doe@example.com', ', ')
- The result will be an array:
[ "John", "Doe", "john.doe@example.com" ]
. - Use the index to extract the desired part:powerautomateCopy code
split('John, Doe, john.doe@example.com', ', ')[2]
Output:john.doe@example.com
.
Practical Application:
- Parsing log data.
- Extracting details from structured input fields in forms.
- Breaking down addresses or product SKUs.
2. Conditional String Processing: Making Decisions Based on Data
The if()
and contains()
functions allow you to process strings conditionally. This is especially useful when dealing with dynamic or inconsistent data.
Example: Validating Email Domains
You want to check if an email address belongs to a specific domain like "@example.com"
.
Steps:
- Use the
contains()
function:powerautomateCopy codecontains('john.doe@example.com', '@example.com')
- Combine with
if()
for conditional logic:powerautomateCopy codeif(contains('john.doe@example.com', '@example.com'), 'Valid', 'Invalid')
Output:Valid
.
Practical Application:
- Validating user input (e.g., phone numbers or email addresses).
- Filtering out irrelevant or unwanted data.
- Enabling dynamic routing in workflows based on string content.
3. Nested Expressions: Combining Functions for Complex Transformations
Power Automate allows you to nest functions, enabling intricate string manipulation. While this can be tricky to read, it’s highly effective for combining multiple operations.
Example: Formatting Names
You have a string "john doe"
and want to convert it to "John Doe"
.
Steps:
- Split the string into an array:powerautomateCopy code
split('john doe', ' ')
Result:[ "john", "doe" ]
. - Capitalize each word:powerautomateCopy code
concat(toUpper(substring(items(0), 0, 1)), substring(items(0), 1), ' ', toUpper(substring(items(1), 0, 1)), substring(items(1), 1))
Output:John Doe
. - Nest the operations in a
join()
to handle dynamic arrays:powerautomateCopy codejoin(apply(variables('NameArray'), concat(toUpper(substring(item(), 0, 1)), substring(item(), 1))), ' ')
Practical Application:
- Formatting text for reports or notifications.
- Generating human-readable content dynamically.
- Advanced data transformation pipelines.
Best Practices for Advanced String Manipulation
- Break Down Complex Logic: Use variables to store intermediate results for readability and debugging.
- Use Inline Comments: Document nested expressions for clarity.
- Optimize for Performance: Avoid unnecessary loops or redundant operations, especially in workflows with large datasets.
- Test Iteratively: Use test inputs to validate each part of your expression to avoid errors.
Conclusion
Mastering advanced string manipulation techniques in Power Automate opens doors to more sophisticated and efficient workflows. By leveraging functions like split()
, if()
, contains()
, and nested operations, you can handle complex data processing scenarios with ease. Whether you’re formatting inputs, parsing logs, or generating dynamic content, these techniques empower you to streamline operations and deliver accurate results.